16.MyBatis配置文件2
使用代理
1.删除Dao的impl包及其实现类
2.Mapper.xml与Dao接口
(1)<mapper namespace="[Dao接口的全限定名]">
(2)<insert id="[Dao接口中的对应方法名]">
1 | package com.course.dao; |
1 |
|
3.测试类
1 | package com.course.test; |
1 | DEBUG [main] - Logging initialized using 'class org.apache.ibatis.logging.slf4j.Slf4jImpl' adapter. |
若<mapper namespace="">
与接口的全限定名不对应:
1 | Exception in thread "main" org.apache.ibatis.binding.BindingException: Type interface com.course.dao.UserDao is not known to the MapperRegistry. |
若Mapper.xml的节点id与接口中的方法名不对应:
1 | Exception in thread "main" org.apache.ibatis.binding.BindingException: Invalid bound statement (not found): com.course.dao.UserDao.getUserById |
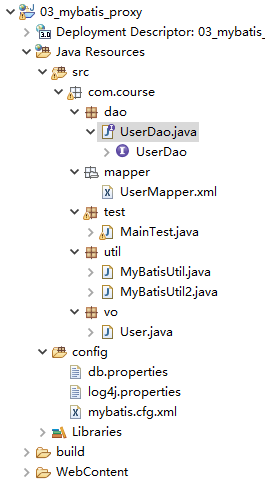
Mapper.xml详解
1 |
|
parameterMap和resultMap的id可以相同
配置别名
mybatis.cfg.xml
1 |
|
mapper.xml
1 |
|
mybatis占位符的处理
1,占位符一:#{xxx}
PreparedStatement预编译sql语句
xxx表达式的写法
- 参数类型为javabean类,xxx表达式必须和javabean中属性对应的get方法名字一样
- 参数类型为简单类型,xxx表达式随表写,保持和参数的名字一致
1 | <mapper namespace="com.course.dao.UserDao"> |
1 | DEBUG [main] - ==> Preparing: insert into user(name, age, address, birthday) values(?, ?, ?, ?) |
2,占位符二:${xxx}
Statement拼接sql语句
xxx表达式的写法
- 参数类型为javabean类,xxx表达式必须和javabean中属性对应的get方法名字一样
- 参数类型为简单类型,xxx表达式执行只能写${value}
1 | <mapper namespace="com.course.dao.UserDao"> |
1 | DEBUG [main] - ==> Preparing: insert into user(name, age, address) values('user18', 10, 'address18') |
1 | <mapper namespace="com.course.dao.UserDao"> |
1 | Exception in thread "main" org.apache.ibatis.exceptions.PersistenceException: |
1 | <mapper namespace="com.course.dao.UserDao"> |
1 | DEBUG [main] - ==> Preparing: select * from user where id=12 |