18.java连接redis
java连接redis
1. Jedis所需要的jar包依赖
1 | <dependency> |
2. Jedis常用操作
2.1 测试连通性
1 | package com.redis.demo; |
2.2 基本操作
1 | package com.redis.demo; |
output:
1 | ----------key---------- |
2.3 事务提交
exec:
1 | package com.redis.demo; |
discard:
1 | package com.redis.demo; |
watch:
1 | package com.redis.demo; |
unwatch:
1 | package com.redis.demo; |
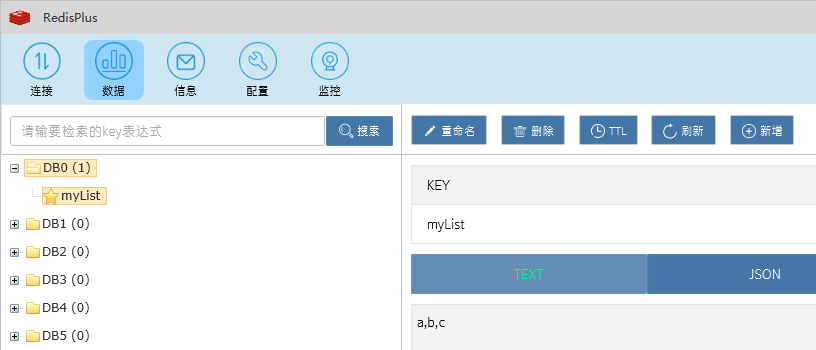
2.4 主从复制
1 | package com.redis.demo; |
1 | jedisSlave.set("number", "200"); |
会抛出以下异常:
1 | Exception in thread "main" redis.clients.jedis.exceptions.JedisDataException: READONLY You can't write against a read only replica. |
2.6 集群连接
1 | [root@localhost redis_cluster]# ps -ef | grep redis |
1 | package com.redis.demo; |
3. JedisPool
3.1 为什么要使用JedisPool
- 1.获取Jedis实例需要从JedisPool中获取
- 2.用完Jedis实例需要返还给JedisPool
- 3.如果Jedis在使用过程中出错,则也需要还给JedisPool
3.2 案例及代码
1 | package com.redis.demo; |
1 | package com.redis.demo; |
3.3 配置总结all
JedisPool
的配置参数大部分是由JedisPoolConfig
的对应项来赋值的。
maxTotal/maxActive: 控制一个pool可分配多少个jedis实例,通过pool.getResource()来获取;如果赋值为-1,则表示不限制;如果pool已经分配了maxActive个jedis实例,则此时pool的状态为exhausted。
maxIdle:控制一个pool最多有多少个状态为idle(空闲)的jedis实例
whenExhaustedAction: 表示当pool中的jedis实例都被allocated完时,pool要采取的操作;默认有三种。
WHEN_EXHAUSTED_FAIL –> 表示无jedis实例时,直接抛出NoSuchElementException;
WHEN_EXHAUSTED_BLOCK –> 则表示阻塞住,或者达到maxWait时抛出JedisConnectionException;
WHEN_EXHAUSTED_GROW –> 则表示新建一个jedis实例,也就说设置的maxActive无用;
setMaxWaitMillis/maxWait: 表示当borrow一个jedis实例时,最大的等待时间,如果超过等待时间,则直接抛JedisConnectionException;
testOnBorrow: 获得一个jedis实例的时候是否检查连接可用性(ping());如果为true,则得到的jedis实例均是可用的
testOnReturn: return 一个jedis实例给pool时,是否检查连接可用性(ping());
testWhileIdle: 如果为true,表示有一个idle object evitor线程对idle object进行扫描,如果validate失败,此object会被从pool中drop掉;这一项只有在timeBetweenEvictionRunsMillis大于0时才有意义;
timeBetweenEvictionRunsMillis: 表示idle object evitor两次扫描之间要sleep的毫秒数;
numTestsPerEvictionRun: 表示idle object evitor每次扫描的最多的对象数;
minEvictableIdleTimeMillis: 表示一个对象至少停留在idle状态的最短时间,然后才能被idle object evitor扫描并驱逐;这一项只有在timeBetweenEvictionRunsMillis大于0时才有意义;
softMinEvictableIdleTimeMillis: 在minEvictableIdleTimeMillis基础上,加入了至少minIdle个对象已经在pool里面了。如果为-1,evicted不会根据idle time驱逐任何对象。如果minEvictableIdleTimeMillis>0,则此项设置无意义,且只有在timeBetweenEvictionRunsMillis大于0时才有意义;
lifo: borrowObject返回对象时,是采用DEFAULT_LIFO(last in first out,即类似cache的最频繁使用队列),如果为False,则表示FIFO队列;
其中JedisPoolConfig对一些参数的默认设置如下:
testWhileIdle=true
minEvictableIdleTimeMills=60000
timeBetweenEvictionRunsMillis=30000
numTestsPerEvictionRun=-1
4. Redis存储Object
可以将Java Object转换为json字符串,然后存入Redis,需要使用对象时,从Redis中取得json字符串,然后转换为Java Object
1 | package com.redis.demo; |